The art of API abstraction
Published 4 months ago
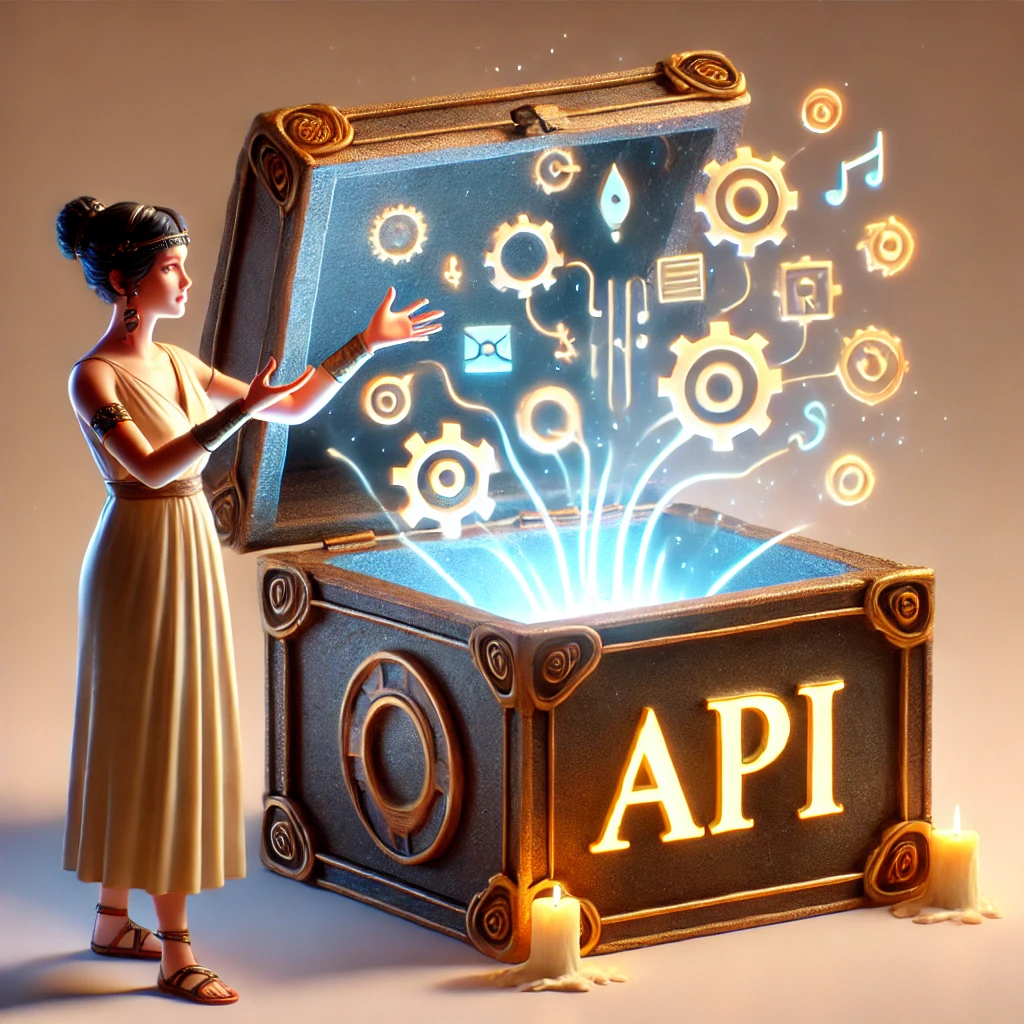
What is an API? (Explain like I’m 5 version)
An API (Application Programming Interface) is like a magic box. You put something in, and something useful comes out—but you don’t have to know how it works inside.
Imagine a vending machine. You press a button, and it gives you candy. You don’t need to know how the machine sorts coins, spins gears, or drops the candy. That’s a black box—you use it without understanding its inner workings.
APIs work the same way. They let different programs talk to each other without revealing the messy details inside.
APIs in real life
APIs exist beyond code. Here are a few examples:
- Driving a car → You don’t need to understand the engine; the steering wheel and pedals are the API.
- Making coffee → A coffee machine is a black box. You press a button, and it does everything for you.
- Restaurants → A menu is an API for ordering food. You don’t need to know how the chef cooks it.
- iPhone vs. Android → Apple’s iPhone has a single button (or none now), abstracting most functionality away. Android, on the other hand, kept the three-button navigation—especially the back button, which gives users more control. Both are APIs, but they strike different balances between abstraction and usability.
The best APIs, in code or in life, simplify complexity. But abstraction is a double-edged sword: abstract too much, and users lose control; abstract too little, and it becomes too complicated.
Two stories of bad API design
1. The spread operator disaster
At a past job, I built an API for event tracking. Initially, it was clean. But then the CTO asked me to use Java’s newly introcuced (at the time) spread operator (...params
) to allow flexible arguments.
Bad idea.
Developers had no clue what to pass. Some added event names, others threw in random metadata. The API became an unpredictable black box—people threw things in, but didn’t know what would come out.
The fix? I introduced an AnalyticsParamsBuilder
, guiding developers with a structured, self-documenting approach.
2. The Fizzle Compiler Confusion
At another job, we had a tool (let’s call it Fizzle) that compiled assets. By default, it compiled everything unless you opted out with flags:
fizzle --exclude images --exclude js
This was backward. If you forgot to exclude something, it got compiled anyway.
We flipped the logic: Fizzle compiled nothing unless explicitly instructed. Now, developers had to opt in:
fizzle --compile images --compile js
or simply:
fizzle --compile all
Suddenly, the API was predictable and user-friendly.
The takeaway
A great API—whether in software or life—hides complexity without sacrificing clarity.
Before designing an API, ask yourself:
- Does it reduce cognitive load?
- Is it predictable and consistent?
- Does it provide the right level of abstraction?
Here are some guidelines I follow whenever I design an API:
1. Be a master of your domain
You cannot build a good API if you don’t deeply understand the domain it serves. Abstractions only make sense when designed by someone who knows what should and shouldn’t be exposed. Learn the pain points of your users before trying to simplify things for them.
2. Abstract the right things
Not everything should be abstracted. If you hide too much, you strip away control and flexibility. If you abstract too little, users end up writing hacks to make things work.
- Example: Allow customization, but within constraints.
- Golden Rule: Never trust the user. If they can break your API, they will. Guardrail them into doing the right thing.
3. Don’t make it too easy
A common mistake is over-simplifying an API to the point where users don’t need to understand it. This leads to lazy usage and bad implementation practices.
- Make users go through your documentation. They should understand how and why your API works.
- Do not enforce shortcuts that promote misuse. If someone uses your API wrong, the blame is on your design.
4. Users will do whatever works for them
Users don’t always care about efficiency or best practices—they care about what works.
- I once worked with people who clean installed our entire project every time they needed to rebuild it. It took 5 minutes instead of manually changing a file, which would take 5 seconds.
- Why?
- Because they were lazy.
- Because they feared the other way might break things.
Your API should guide users toward the right way, because left unchecked, they will default to whatever is easiest for them.
5. Make things easy, but not too easy
Users don’t want to know how your API works under the hood. They expect it to work.
- A car has a steering wheel instead of individual controls for each tire.
- A coffee machine doesn’t require you to manually adjust water temperature.
APIs should follow the same principle—hide complexity, but don’t strip away control.
The Balance of API Design
A good API is a well-designed black box—you know what it does, you trust how it works, and you don’t need to open it.
Just like a vending machine, a car’s controls, or a coffee machine, the best APIs are useful black boxes—they just work.
And abstracting the right things on an API is an art.